什么是JavaScriptExecutor?
JavaScriptExecutor是一个通过Selenium Webdriver帮助执行JavaScript的接口。JavaScriptExecutor提供了两个方法“ecutescript”和“ecuteAsyncScript”来在选定的窗口或当前页面上运行javascript。
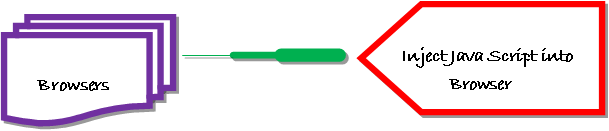
在本教程中,将了解-
- 什么是JavaScriptExecutor?
- 我们为什么需要JavaScriptExecutor?
- JavaScriptExecutor方法
-
ecuteAsyncScript示例
- 示例1:在被测浏览器中执行休眠。
-
执行脚本示例
- 1)示例:点击按钮登录并生成预警窗口
- 2)示例:捕获抓取数据并导航到不同页面
- 3)示例:向下滚动
我们为什么需要JavaScriptExecutor?
在Selenium Webdriver中,XPath、CSS等定位器用于标识和执行对网页的操作。
如果这些定位器不起作用,可以使用JavaScriptExecutor。可以使用JavaScriptExecutor对Web元素执行所需的操作。
Selenium支持javaScriptExecutor。要使用JavaScriptExecutor,只需在脚本中导入(org.openqa.selenium.JavascriptExecutor)就可以了。
JavaScriptExecutor方法
- ecuteAsyncScript
使用异步脚本,页面呈现速度更快。这样执行的JS不是强制用户,而是带有同步运行的各种回调函数的单线程。
- 执行脚本
此方法在Selenium中当前选定的框架或窗口的上下文中执行JavaScript。我们还可以将复杂的参数传递给它。
该脚本可以返回值。返回的数据类型为
- Boolean
- Long
- String
- List
- WebElement
JavascriptExecutor的基本语法如下:
语法:
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript(Script,Arguments);
- 脚本-这是需要执行的JavaScript。
- 参数-它是脚本的参数。这是可选的。
ecuteAsyncScript示例
使用ecuteAsyncScript有助于提高测试的性能。它允许编写更像普通编码的测试。
execSync会阻止Selenium浏览器执行的进一步操作,但execAsync不会阻止挡路操作。一旦脚本完成,它将向服务器端测试套件发送回调。这意味着脚本中的所有内容都将由浏览器而不是服务器执行。
示例1:在被测浏览器中执行休眠。
在这个场景中,我们将使用“Guru99”演示站点来演示ecuteAsyncScript。在此示例中,将
- 启动浏览器。
- 打开站点“http://www.itxiaonv.com/V4/”。
- 应用程序等待5秒以执行进一步操作。
步骤1)使用 executeAsyncScript() 方法捕获等待5秒(5,000毫秒)前的开始时间。
步骤2)然后,使用 executeAsyncScript() 等待5秒。
步骤3)然后,获取当前时间。
步骤4)减去(当前时间-开始时间)=经过的时间。
步骤5)验证应显示5000毫秒以上的输出
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.testng.annotations.Test;
public class JavaSE_Test {
@Test
public void Login() {
WebDriver driver= new FirefoxDriver();
//Creating the JavascriptExecutor interface object by Type casting
JavascriptExecutor js = (JavascriptExecutor)driver;
//Launching the Site.
driver.get("http://www.itxiaonv.com/V4/");
//Maximize window
driver.manage().window().maximize();
//Set the Script Timeout to 20 seconds
driver.manage().timeouts().setScriptTimeout(20, TimeUnit.SECONDS);
//Declare and set the start time
long start_time = System.currentTimeMillis();
//Call executeAsyncScript() method to wait for 5 seconds
js.executeAsyncScript("window.setTimeout(arguments[arguments.length - 1], 5000);");
//Get the difference (currentTime - startTime) of times.
System.out.println("Passed time: " + (System.currentTimeMillis() - start_time));
}
}
输出:成功显示超过5 seconds(5000 miliseconds) 的流逝时间,如下图所示:
[TestNG] Running:
C:
\Users\gauravn\AppData\Local\Temp\testng-eclipse-387352559\testng-customsuite.xml
log4j:
WARN No appenders could be found for logger (org.apache.http.client.protocol.RequestAddCookies).
log4j:
WARN Please initialize the log4j system properly.
log4j:
WARN See http://logging.apache.org/log4j/1.2/faq.html#noconfig for more info.
Passed time: 5022
PASSED:
Login
===============================================
Default test
Tests run: 1, Failures: 0, Skips: 0
===============================================
执行脚本示例
对于ecuteScript,我们将逐一看到三个不同的示例。
1)示例:点击按钮登录,使用JavaScriptExecutor生成预警窗口。
在这个场景中,我们将使用“Guru99”演示站点来演示JavaScriptExecutor。在此示例中,
- 启动Web浏览器
- 打开站点“http://www.itxiaonv.com/V4/”并
- 使用凭据登录
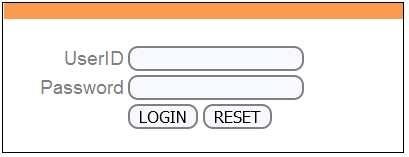
- 在成功登录时显示警告窗口。
import org.openqa.selenium.By;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.testng.annotations.Test;
public class JavaSE_Test {
@Test
public void Login() {
WebDriver driver= new FirefoxDriver();
//Creating the JavascriptExecutor interface object by Type casting
JavascriptExecutor js = (JavascriptExecutor)driver;
//Launching the Site.
driver.get("http://www.itxiaonv.com/V4/");
WebElement button =driver.findElement(By.name("btnLogin"));
//Login to Guru99
driver.findElement(By.name("uid")).sendKeys("mngr34926");
driver.findElement(By.name("password")).sendKeys("amUpenu");
//Perform Click on LOGIN button using JavascriptExecutor
js.executeScript("arguments[0].click();", button);
//To generate Alert window using JavascriptExecutor. Display the alert message
js.executeScript("alert('Welcome to Guru99');");
}
}
输出:当代码成功执行时。会观察到
- 成功单击登录按钮
- 将显示警告窗口(见下图)。
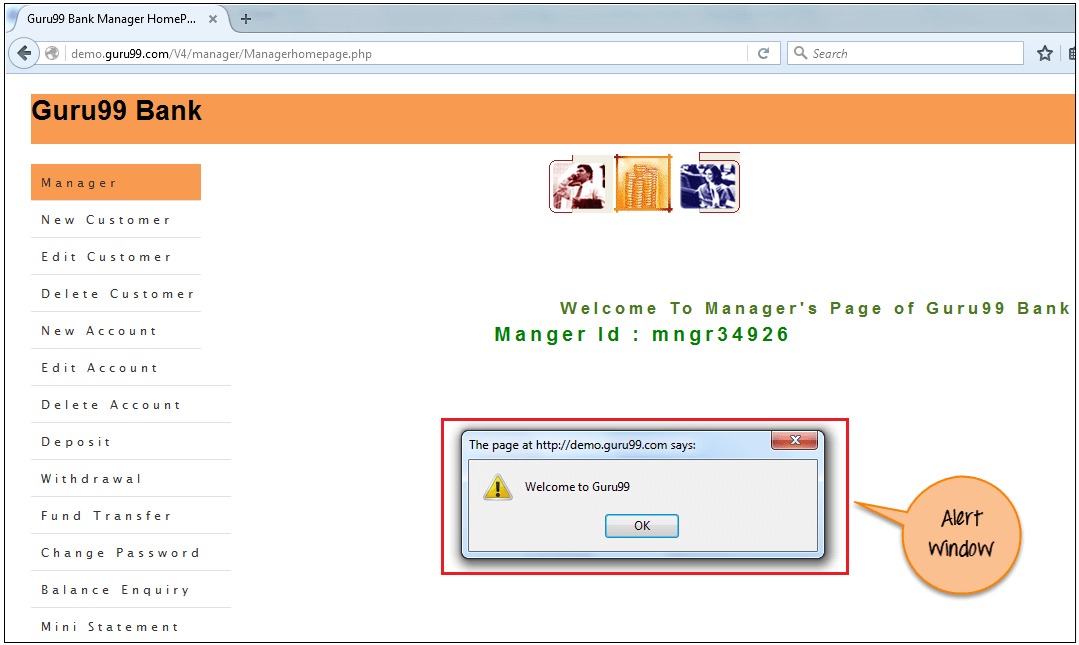
2)示例:使用JavaScriptExecutor捕获抓取数据并导航到不同的页面。
执行以下Selenium脚本。在此示例中,
- 启动网站
- 获取网站的详细信息,如网站的URL、网站的标题名称和域名。
- 然后导航到不同的页面。
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.testng.annotations.Test;
public class JavaSE_Test {
@Test
public void Login() {
WebDriver driver= new FirefoxDriver();
//Creating the JavascriptExecutor interface object by Type casting
JavascriptExecutor js = (JavascriptExecutor)driver;
//Launching the Site.
driver.get("http://www.itxiaonv.com/V4/");
//Fetching the Domain Name of the site. Tostring() change object to name.
String DomainName = js.executeScript("return document.domain;").toString();
System.out.println("Domain name of the site = "+DomainName);
//Fetching the URL of the site. Tostring() change object to name
String url = js.executeScript("return document.URL;").toString();
System.out.println("URL of the site = "+url);
//Method document.title fetch the Title name of the site. Tostring() change object to name
String TitleName = js.executeScript("return document.title;").toString();
System.out.println("Title of the page = "+TitleName);
//Navigate to new Page i.e to generate access page. (launch new url)
js.executeScript("window.location = 'http://www.itxiaonv.com/'");
}
}
输出:当上述代码成功执行时,它将获取站点的详细信息并导航到不同的页面,如下所示。
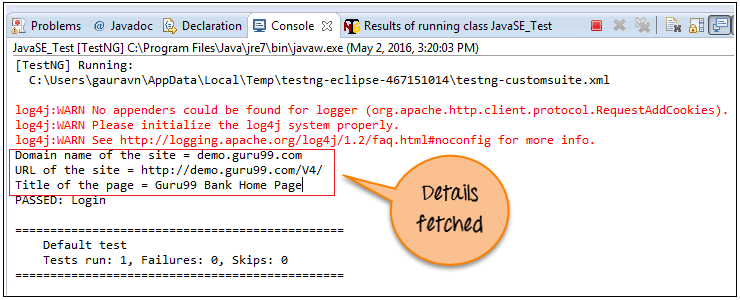
[TestNG] Running:
C:
\Users\gauravn\AppData\Local\Temp\testng-eclipse-467151014\testng-customsuite.xml
log4j:
WARN No appenders could be found for logger (org.apache.http.client.protocol.RequestAddCookies).
log4j:
WARN Please initialize the log4j system properly.
log4j:
WARN See http://logging.apache.org/log4j/1.2/faq.html#noconfig for more info.
Domain name of the site = www.itxiaonv.com
URL of the site = http://www.itxiaonv.com/V4/
Title of the page = Guru99 Bank Home Page
PASSED:
Login
===============================================
Default test
Tests run: 1, Failures: 0, Skips: 0
===============================================
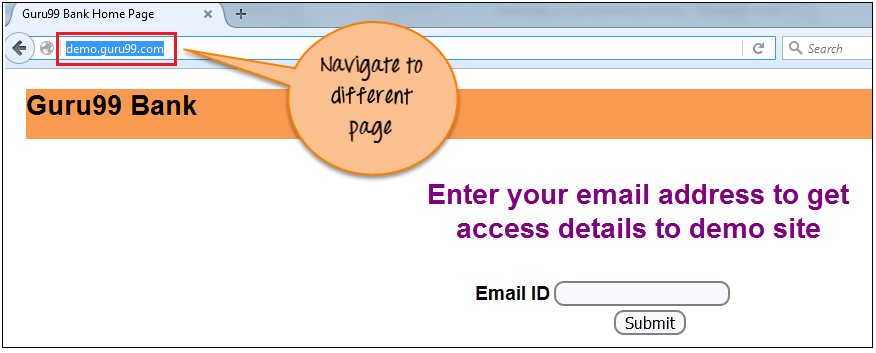
3)示例:使用JavaScriptExecutor向下滚动。
执行以下Selenium脚本。在此示例中,
- 启动网站
- 向下滚动600像素
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.testng.annotations.Test;
public class JavaSE_Test {
@Test
public void Login() {
WebDriver driver= new FirefoxDriver();
//Creating the JavascriptExecutor interface object by Type casting
JavascriptExecutor js = (JavascriptExecutor)driver;
//Launching the Site.
driver.get("http://moneyboats.com/");
//Maximize window
driver.manage().window().maximize();
//Vertical scroll down by 600 pixels
js.executeScript("window.scrollBy(0,600)");
}
}
输出:当执行上述代码时,它将向下滚动600像素(见下图)。
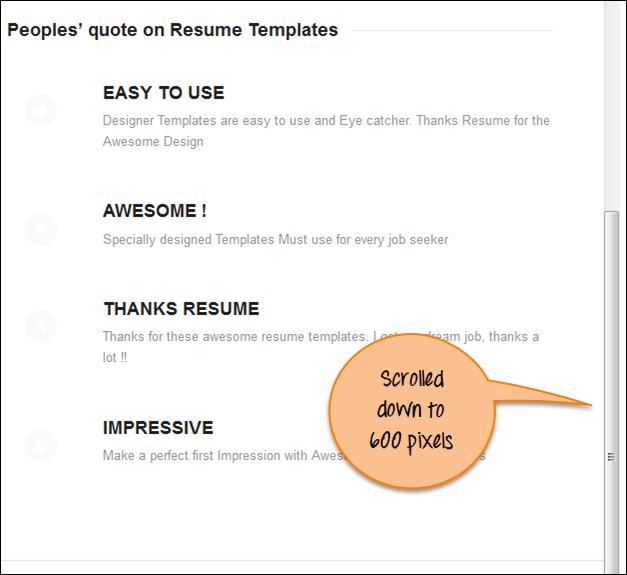
总结:
当Selenium Webdriver由于某些问题而无法单击任何元素时,将使用JavaScriptExecutor。
- JavaScriptExecutor提供了两个方法“ecutescript”和“ecuteAsyncScript”来处理。
- 使用Selenium Webdriver执行JavaScript。
- 演示了如果Selenium由于某些问题无法单击元素,如何通过JavaScriptExecutor单击元素。
- 使用JavaScriptExecutor生成了“Alert”窗口。
- 使用JavaScriptExecutor导航到不同的页面。
- 已使用JavaScriptExecutor向下滚动窗口。
- 使用JavaScriptExecutor获取的URL、标题和域名。