在本教程中,将了解-
- 如何使用if语句
- 不同类型的条件语句
- if语句
- if…else语句
- if…else语句
如何使用条件语句
条件语句用于根据不同的条件决定执行流程。如果条件为真,则可以执行一个操作;如果条件为假,则可以执行另一个操作。
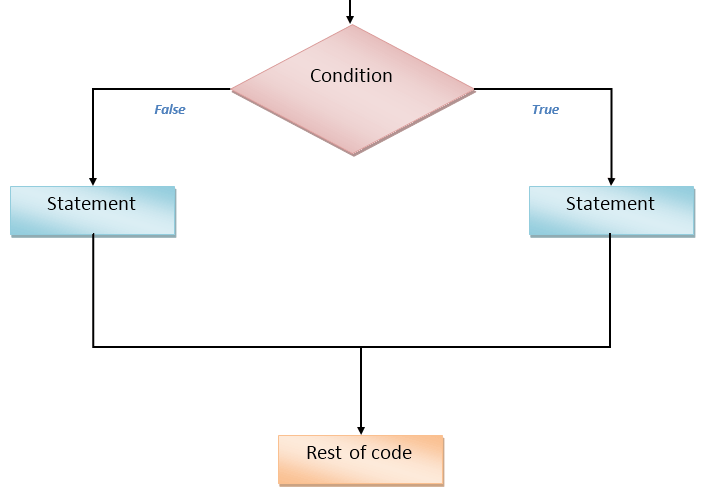
不同类型的条件语句
JavaScript中主要有三种类型的条件语句。
- If statement
- If…else statement
- If…else If…else statement
if语句
语法:
如果只想检查特定条件,可以使用If语句。
自己试试这个:
<html>
<head>
<title>
IF Statments!!!
</title>
<script type="text/javascript">
var age = prompt("Please enter your age");
if(age>=18)
document.write("You are an adult <br />");
if(age<18)
document.write("You are NOT an adult <br />");
</script>
</head>
<body>
</body>
</html>
if…else语句
语法:
if (condition)
{
lines of code to be executed if the condition is true
}
else
{
lines of code to be executed if the condition is false
}
可以使用if….else语句(如果必须检查两个条件并执行一组不同的代码)。
自己试试这个:
<html>
<head>
<title>
if...else statments!!!
</title>
<script type="text/javascript">
// Get the current hours
var hours = new Date().getHours();
if(hours<12)
document.write("Good Morning!!!<br />");
else
document.write("Good Afternoon!!!<br />");
</script>
</head>
<body>
</body>
</html>
if…else if…else
语法:
if (condition1)
{
lines of code to be executed if condition1 is true
}
else if(condition2)
{
lines of code to be executed if condition2 is true
}
else
{
lines of code to be executed if condition1 is false and condition2 is false
}
可以使用if….else if…如果要检查两个以上的条件,使用else语句。
自己试试这个:
<html>
<head>
<script type="text/javascript">
var one = prompt("Enter the first number");
var two = prompt("Enter the second number");
one = parseInt(one);
two = parseInt(two);
if (one == two)
document.write(one + " is equal to " + two + ".");
else if (one<two)
document.write(one + " is less than " + two + ".");
else
document.write(one + " is greater than " + two + ".");
</script>
</head>
<body>
</body>
</html>